[PHP] 문자열 AES-256-CBC 암복호화
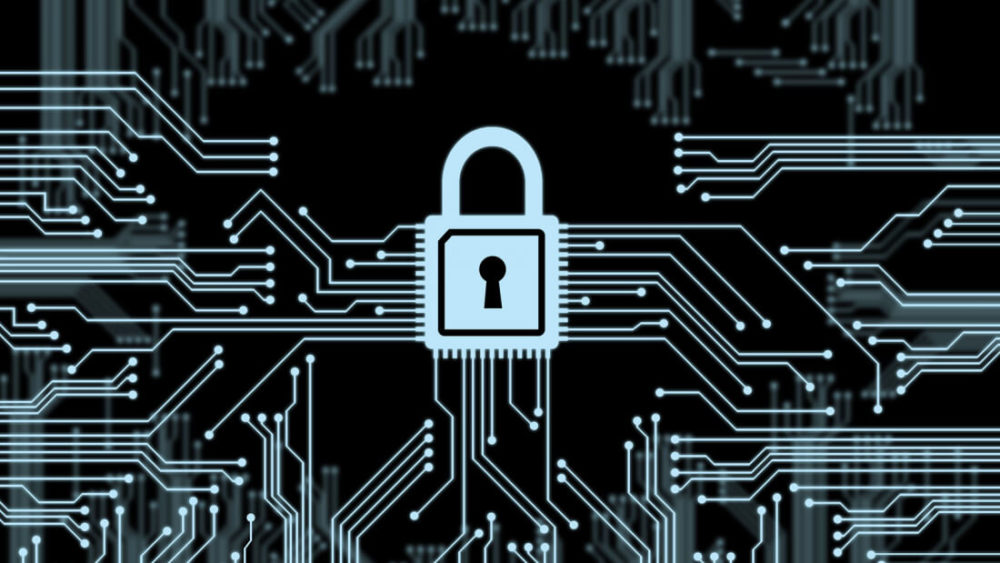
개발 작업을 진행하다보면 보안을 위해 문자열을 암호화해서 보관하고 필요 때 복호화해서 사용하는 경우가 많다. 비밀번호 같은 경우는 복호화가 불가능하도록 암호화를 하지만 다른 서비스의 로그인 정보 등은 서비스 접근 때 사용되어야 하기 때문에 복호화할 수 있어야 한다. 그럴 때 사용할 수 있는 AES-256-CBC
암복호화 함수를 간단하게 만들었다. PHP openssl
확장을 이용하는 것으로 해당 모듈이 설치되어 있지 않다면 사용이 불가능 하다.
데모 : http://demo.chicpro.dev/aes256.php
<?php
define('SALT', 'zvJc5@77+uC!5#F*dGMysvjRGP7M25G$F6!VzF=y');
function AESEncrypt($str, $key = '')
{
if (!$key)
$key = SALT;
return base64_encode(openssl_encrypt($str, "AES-256-CBC", $key, true, str_repeat(chr(0), 16)));
}
function AESDecrypt($str, $key = '')
{
if (!$key)
$key = SALT;
return openssl_decrypt(base64_decode($str), "AES-256-CBC", $key, true, str_repeat(chr(0), 16));
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AES 암복화 Demo</title>
</head>
<body>
<form name="faes" method="get">
<p>
<label for="word">단어</label>
<input type="text" name="word" id="word" maxlength="50">
</p>
<p>
<input type="submit" value="암호화">
</p>
</form>
<?php if (isset($_GET['word']) && $_GET['word']) { ?>
<p>
암호화 문자열 : <?php echo $str = AESEncrypt($_GET['word']); ?>
</p>
<form name="faes" method="get">
<p>
<label for="str">암호화 문자열</label>
<input type="text" name="str" id="str" value="<?php echo $str; ?>">
</p>
<p>
<input type="submit" value="복호화">
</p>
</form>
<?php } ?>
<?php if (isset($_GET['str']) && $_GET['str']) { ?>
<p>
복호화 단어 : <?php echo AESDecrypt($_GET['str']); ?>
</p>
<?php } ?>
</body>
</html>
위 코드에서 상수 SALT
값은 외부에 노출되어선 안된다. 암호화된 데이터를 복호화할 수 있기 때문이다.